The Tinify API allows you to compress and optimize AVIF, WebP, JPEG and PNG images. It is designed as a REST service. The client libraries in various languages make it very easy to interact with the Tinify API.
Installation
You can use the Node.js client by installing the npm package and adding it to your application’s dependencies:
npm install --save tinify
The source code is available on Github.
Authentication
To use the API you must provide your API key. You can get an API key by registering with your name and email address. Always keep your API key secret!
const tinify = require("tinify");
tinify.key = "YOUR_API_KEY";
All requests will be made over an encrypted HTTPS connection.
You can instruct the API client to make all requests over an HTTP proxy. Set the URL of your proxy server, which can optionally include credentials.
tinify.proxy = "http://user:pass@192.168.0.1:8080";
Compressing images
You can upload any AVIF, WebP, JPEG or PNG image to the Tinify API to compress it. We will automatically detect the type of image and optimise with the TinyPNG or TinyJPG engine accordingly. Compression will start as soon as you upload a file or provide the URL to the image.
You can choose a local file as the source and write it to another file.
const source = tinify.fromFile("unoptimized.webp");
source.toFile("optimized.webp");
You can also upload an image from a buffer (a string with binary) and get the compressed image data.
const fs = require("fs");
fs.readFile("unoptimized.jpg", function(err, sourceData) {
if (err) throw err;
tinify.fromBuffer(sourceData).toBuffer(function(err, resultData) {
if (err) throw err;
// ...
});
});
You can provide a URL to your image instead of having to upload it.
const source = tinify.fromUrl("https://tinypng.com/images/panda-happy.png");
source.toFile("optimized.png");
Resizing images
Use the API to create resized versions of your uploaded images. By letting the API handle resizing you avoid having to write such code yourself and you will only have to upload your image once. The resized images will be optimally compressed with a nice and crisp appearance.
You can also take advantage of intelligent cropping to create thumbnails that focus on the most visually important areas of your image.
Resizing counts as one additional compression. For example, if you upload a single image and retrieve the optimized version plus 2 resized versions this will count as 3 compressions in total.
To resize an image, call the resize
method on an image source:
const source = tinify.fromFile("large.jpg");
const resized = source.resize({
method: "fit",
width: 150,
height: 100
});
resized.toFile("thumbnail.jpg");
The method
describes the way your image will be resized. The following
methods are available:
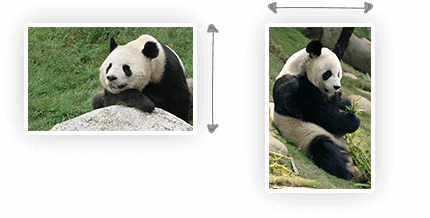
scale
-
Scales the image down proportionally. You must provide either a target
width
or a targetheight
, but not both. The scaled image will have exactly the provided width or height.
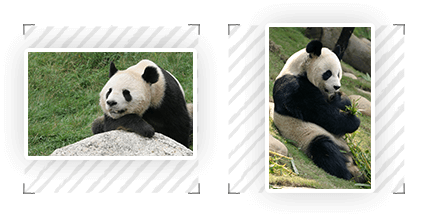
fit
-
Scales the image down proportionally so that it fits within the given
dimensions. You must provide both a
width
and aheight
. The scaled image will not exceed either of these dimensions.
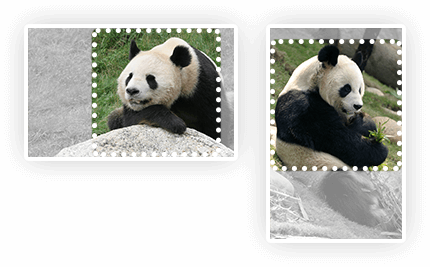
cover
-
Scales the image proportionally and crops it if necessary so that
the result has exactly the given dimensions. You must provide both a
width
and aheight
. Which parts of the image are cropped away is determined automatically. An intelligent algorithm determines the most important areas of your image.
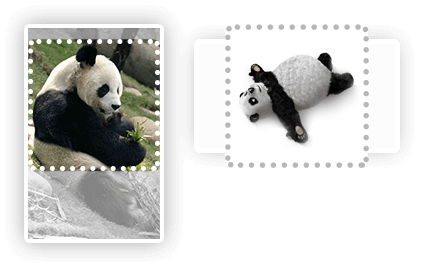
thumb
-
A more advanced implementation of cover that also detects cut out
images with plain backgrounds. The image is scaled down to the
width
andheight
you provide. If an image is detected with a free standing object it will add more background space where necessary or crop the unimportant parts. This feature is new and we’d love to hear your feedback!
If the target dimensions are larger than the original dimensions, the image will not be scaled up. Scaling up is prevented in order to protect the quality of your images.
Converting images
You can use the API to convert your images to your desired image type. Tinify currently supports converting between AVIF, WebP, JPEG, and PNG. When you provide more than one image type in your convert request, the smallest version will be returned to you.
Image converting will count as one additional compression.
const source = tinify.fromFile("panda-sticker.jpg");
const converted = source.convert({type:["image/webp","image/png"]});
const extension = converted.result().extension();
extension.then(ext => {
converted.toFile("panda-sticker." + ext);
})
Images with transparency
Images with a transparent background will only be converted to formats that support transparency. In case you want to include formats that do not support transparency (JPEG), one can specify a background color that replaces the transparency using the transform object.
If you wish to convert an image with a transparent background to one with a solid background,
specify a background
property in the transform
object. If this property is provided,
the background of a transparent image will be filled.
const source = tinify.fromFile("panda-sticker.png");
const converted = source.convert({type:"image/jpeg"}).transform({background:"#000000"});
converted.toFile("panda-sticker.jpg");
Request options
You can provide the following options to convert your image:
convert
-
Your desired image types. The following options are available as a
type
:-
One image type, specified as a string
"image/webp"
. -
Multiple image types, specified as an array
["image/webp","image/png"]
. The smallest of the provided image types will be returned. -
The wildcard
"*/*"
returns the smallest of Tinify's supported image types, currently AVIF, WebP, JPEG and PNG.
-
One image type, specified as a string
transform
-
The transform object specifies the stylistic transformations that will be applied to your image.
Include a
background
property to fill a transparent image's background. The following options are available to specify a background color:-
A hex value. Custom background color using the color's hex value:
"#000000"
. -
"white"
or"black"
. Only the colors white and black are supported as strings.
-
A hex value. Custom background color using the color's hex value:
Preserving metadata
You can request that specific metadata is copied from the uploaded image
to the compressed version. Preserving copyright
information, the GPS
location
and the creation
date are currently supported. Preserving
metadata adds to the compressed file size, so you should only preserve
metadata that is important to keep.
Preserving metadata will not count as an extra compression. However, in the background the image will be created again with the additional metadata.
To preserve specific metadata, call the preserve
method on an image
source:
const source = tinify.fromFile("large.jpg");
const copyrighted = source.preserve("copyright", "creation");
copyrighted.toFile("optimized-copyright.jpg");
You can provide the following options to preserve specific metadata. No metadata will be added if the requested metadata is not present in the uploaded image.
copyright
- Preserves any copyright information. This includes the EXIF copyright tag (JPEG), the XMP rights tag (PNG) as well as a Photoshop copyright flag or URL. Uses up to 90 additional bytes, plus the length of the copyright data.
creation
- Preserves any creation date or time. This is the moment the image or photo was originally created. This includes the EXIF original date time tag (JPEG) or the XMP creation time (PNG). Uses around 70 additional bytes.
location
(JPEG only)- Preserves any GPS location data that describes where the image or photo was taken. This includes the EXIF GPS latitude and GPS longitude tags (JPEG). Uses around 130 additional bytes.
Saving to Amazon S3
You can ask the Tinify API to save compressed images straight to your Amazon S3. This feature is useful if you use S3 for images hosting, eliminating the need to download them to your server and manually upload them to S3. Each upload will be counted as an additional compression.
To save an image to S3, call the store
method on an image source:
const source = tinify.fromFile("large.jpg");
source.store({
service: "s3",
aws_access_key_id: "AKIAIOSFODNN7EXAMPLE",
aws_secret_access_key: "wJalrXUtnFEMI/K7MDENG/bPxRfiCYEXAMPLEKEY",
region: "us-west-1",
headers: {
"Cache-Control": "public, max-age=31536000"
},
path: "example-bucket/my-images/optimized.jpg"
});
You need to provide the following options in order to save an image on Amazon S3:
service
-
Specify
s3
to store to Amazon S3. aws_access_key_id
aws_secret_access_key
- Your AWS access key ID and secret access key. These are the credentials to an Amazon AWS user account. Find out how to obtain them in Amazon’s documentation. The user must have the correct permissions, see below for details.
region
- The AWS region in which your S3 bucket is located.
path
-
The path at which you want to store the image including the bucket
name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
.
The following settings are optional:
headers
(experimental)-
You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs.
acl
(optional)-
Set an optional Access Control List (ACL) value to manage object accessibility.
By default, objects are set to
public-read
. Useno-acl
to prevent sending an ACL. For more information about ACL options, refer to the AWS Canned ACL documentation.
service
-
Specify
gcs
to store to Google Cloud Storage. gcp_access_token
- The access token for authenticating to Google's Cloud Platform. Find out how to generate these tokens with the example above.
path
-
The path at which you want to store the image including the bucket
name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
. headers
(experimental)-
You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs. AccountError
- There was a problem with your API key or with your API account. Your request could not be authorized. If your compression limit is reached, you can wait until the next calendar month or upgrade your subscription. After verifying your API key and your account status, you can retry the request. In case of a rate limit exceeded error, please add some delay to consecutive requests. Tinify has a generous rate limit to ensure a high quality of service to all clients.
ClientError
- The request could not be completed because of a problem with the submitted data. The exception message will contain more information. You should not retry the request.
ServerError
- The request could not be completed because of a temporary problem with the Tinify API. It is safe to retry the request after a few minutes. If you see this error repeatedly for a longer period of time, please contact us.
ConnectionError
- The request could not be sent because there was an issue connecting to the Tinify API. You should verify your network connection. It is safe to retry the request.
The user that corresponds to your AWS access key ID must have the
PutObject
and PutObjectAcl
permissions on the paths of the objects you
intend to create.
Example S3 access policy
If you want to create a user with limited access specifically for the Tinify API, you can use the following example policy as a starting point:
{
"Statement": {
"Effect": "Allow",
"Action": [
"s3:PutObject",
"s3:PutObjectAcl"
],
"Resource": [
"arn:aws:s3:::example-bucket/*"
]
}
}
Saving to Google Cloud Storage
You can tell the Tinify API to save compressed images directly to Google Cloud Storage. If you use GCS to host your images this saves you the hassle of downloading images to your server and uploading them to GCS yourself. Each upload will be counted as an additional compression.
Before you can store an image in GCS you will need to generate an access token with a service account.
const {auth} = require("google-auth-library");
async function main() {
const client = await auth.getClient({
scopes: "https://www.googleapis.com/auth/devstorage.read_write",
});
const gcpAccessToken = (await client.getAccessToken()).token;
}
main().catch(console.error);
Once you have generated the access token you can then save the optimised
image directly to GCS by calling the store
method on an image source:
const source = tinify.fromFile("large.jpg");
source.store({
service: "gcs",
gcp_access_token: "EXAMPLE_TOKEN_Ag_0HvsglgriGh5_ZmgUBmjaoGU_EXAMPLE_TOKEN",
headers: {
Cache-Control: "public, max-age=31536000"
},
path: "example-bucket/my-images/optimized.jpg"
});
You need to provide the following options in order to save an image on Google Cloud Storage:
The following settings are optional:
Saving to S3 compatible storage
Although the TinyPNG API does not offer an integrated solution for saving the images directly to S3 compatible storage providers other than Amazon, you can still save your images using the example for your chosen development language.
The S3 API is used by storage providers like Digital Ocean Spaces, Backblaze, G-Core Storage, Wasabi, Century Link Object Storage, Filebase and many others.
If you don’t have the AWS SDK installed already, you can add it using the following command:
npm install --save @aws-sdk/client-s3
Store example
let source = tinify.fromUrl("https://tinypng.com/images/panda-happy.png");
let optimisedImage = source.toBuffer();
const s3Client = new s3.S3({
endpoint: "https://YOUR-ENDPOINT.com",
region: "REGION",
credentials: {
accessKeyId: "KEY_EXAMPLE",
secretAccessKey: "YOUR_SECRET_KEY",
},
});
s3Client.send(new s3.PutObjectCommand({
Bucket: "bucket-name",
Key: "file-name.png",
Body: optimisedImage,
ContentType: "image/png",
}));
The detailed documentation on how to use the S3 API can be found here.
Saving to Microsoft Azure
Although the TinyPNG API does not offer an integrated solution for saving the images directly to Microsoft Azure storage, you can still save your images using the example for your chosen development language.
Keep in mind you will need to install the Azure Storage package using the following command:
npm install --save @azure/storage-blob
Store example
The following example explains how to connect to your blob container and upload your image, using your Azure storage connection string. Your connection string can be found on your Azure storage dashboard.
const connectionString = "<YOUR_AZURE_STORAGE_CONNECTION_STRING>";
const blobServiceClient = BlobServiceClient.fromConnectionString(connectionString);
const containerName = "<YOUR_CONTAINER_NAME>";
const containerClient = blobServiceClient.getContainerClient(containerName);
const source = tinify.fromUrl("https://tinypng.com/images/panda-happy.png");
const buff = await source.toBuffer();
const optimizedImage = "tiny-optimized.png";
const blockBlobClient = containerClient.getBlockBlobClient(optimizedImage);
const uploadBlobResponse = await blockBlobClient.upload(buff, buff.length);
The detailed documentation on how to use the Azure storage API can be found here.
Error handling
The Tinify API uses HTTP status codes to indicate success or failure. Any HTTP errors are converted into error objects, which are passed as the first argument to callback functions.
There are four distinct types of errors. The exception message will contain a more detailed description of the error condition.
You can handle each type of error separately:
tinify.fromFile("unoptimized.jpg").toFile("optimized.jpg", function(err) {
if (err instanceof tinify.AccountError) {
console.log("The error message is: " + err.message);
// Verify your API key and account limit.
} else if (err instanceof tinify.ClientError) {
// Check your source image and request options.
} else if (err instanceof tinify.ServerError) {
// Temporary issue with the Tinify API.
} else if (err instanceof tinify.ConnectionError) {
// A network connection error occurred.
} else {
// Something else went wrong, unrelated to the Tinify API.
}
});
If you are writing code that uses an API key configured by your users, you may want to validate the API key before attempting to compress images. The validation makes a dummy request to check the network connection and verify the API key. An error is thrown if the dummy request fails.
tinify.key = "YOUR_API_KEY";
tinify.validate(function(err) {
if (err) throw err;
// Validation of API key failed.
})
Compression count
The API client automatically keeps track of the number of compressions you have made this month. You can get the compression count after you have validated your API key or after you have made at least one compression request.
let compressionsThisMonth = tinify.compressionCount;
Need help? Got feedback?
We’re always here to help, so if you’re stuck just drop us a note on support@tinify.com. It’s also the perfect place to send us all your suggestions and feedback.