The Tinify API allows you to compress and optimize AVIF, WebP, JPEG and PNG images. It is designed as a REST service. This page describes the raw HTTP API. We also maintain client libraries in various languages that make it very easy to interact with the Tinify API.
The API is hosted at api.tinify.com
.
Authentication
To use the API you must provide your API key. You can get an API key by registering with your name and email address. Always keep your API key secret!
Authentication to the API is done with
HTTP Basic Auth.
All requests require an Authorization
header that contains a Base64
digest of the authentication string api:YOUR_API_KEY
where
YOUR_API_KEY
is the key that can be found on your API account page.
All requests must be made over an encrypted HTTPS connection.
Compressing images
You can upload any AVIF, WebP, JPEG or PNG image to the Tinify API to compress it. We will automatically detect the type of image and optimise with the TinyPNG or TinyJPG engine accordingly. Compression will start as soon as you upload a file or provide the URL to the image.
Example upload from file
You can upload a local file. The post data contains the image binary.
POST /shrink HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
[binary]
HTTP/1.1 201 Created
Compression-Count: 1
Location: https://api.tinify.com/output/2xnsp7jn34e5
Content-Type: application/json
{
"input": {
"size": 207565,
"type": "image/jpeg"
}
}
For example, if you have a file named unoptimized.jpg
in the current
directory:
curl https://api.tinify.com/shrink \
--user api:YOUR_API_KEY \
--data-binary @unoptimized.jpg \
--dump-header /dev/stdout
Example upload from URL
You can also provide a URL to your image instead of having to upload it.
The API accepts a JSON body with the image URL as a source
location.
POST /shrink HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"source": {
"url": "https://tinypng.com/images/panda-happy.png"
}
}
HTTP/1.1 201 Created
Compression-Count: 1
Location: https://api.tinify.com/output/cciutldm823k9e9r
Content-Type: application/json
{
"output": {
"size": 30734,
"type": "image/png"
}
}
For example, if you have https://tinypng.com/images/panda-happy.png
as your source:
curl https://api.tinify.com/shrink \
--user api:YOUR_API_KEY \
--header "Content-Type: application/json" \
--data '{"source": {"url": "https://tinypng.com/images/panda-happy.png"} }' \
--dump-header /dev/stdout
Example download request
The compressed image can be retrieved by using the URL in the Location
header from the previous step.
GET /output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
HTTP/1.1 200 OK
Compression-Count: 1
Image-Width: 530
Image-Height: 300
Content-Type: image/jpeg
Content-Length: 46480
[binary]
For example, you can save the compressed image to a file named
optimized.jpg
and open it:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--output optimized.jpg
open optimized.jpg
Resizing images
Use the API to create resized versions of your uploaded images. By letting the API handle resizing you avoid having to write such code yourself and you will only have to upload your image once. The resized images will be optimally compressed with a nice and crisp appearance.
You can also take advantage of intelligent cropping to create thumbnails that focus on the most visually important areas of your image.
Resizing counts as one additional compression. For example, if you upload a single image and retrieve the optimized version plus 2 resized versions this will count as 3 compressions in total.
Example resize request
You can resize an image by using the URL that was returned in the
Location
header after compressing. A JSON request body has to be
provided together with a Content-Type: application/json
header.
POST /output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"resize": {
"method": "fit",
"width": 150,
"height": 100
}
}
HTTP/1.1 200 OK
Compression-Count: 2
Content-Type: image/jpeg
Image-Width: 150
Image-Height: 85
Content-Length: 12594
[binary]
For example, to save the resized and compressed image to a file named
thumbnail.jpg
:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--header "Content-Type: application/json" \
--data '{ "resize": { "method": "fit", "width": 150, "height": 100 } }' \
--dump-header /dev/stdout --silent \
--output thumbnail.jpg
Request options
The method
describes the way your image will be resized. The following
methods are available:
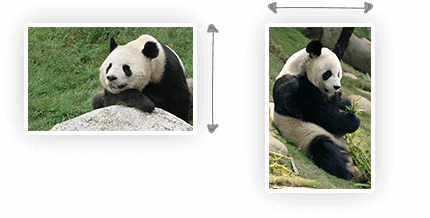
scale
-
Scales the image down proportionally. You must provide either a target
width
or a targetheight
, but not both. The scaled image will have exactly the provided width or height.
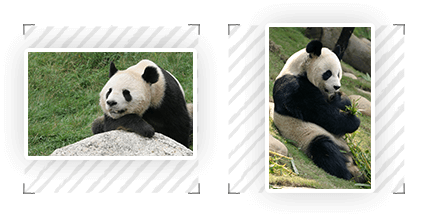
fit
-
Scales the image down proportionally so that it fits within the given
dimensions. You must provide both a
width
and aheight
. The scaled image will not exceed either of these dimensions.
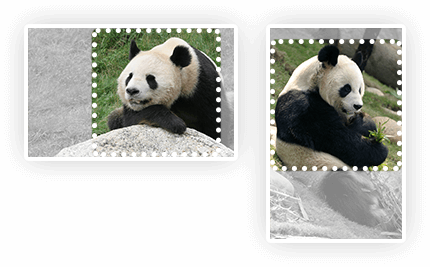
cover
-
Scales the image proportionally and crops it if necessary so that
the result has exactly the given dimensions. You must provide both a
width
and aheight
. Which parts of the image are cropped away is determined automatically. An intelligent algorithm determines the most important areas of your image.
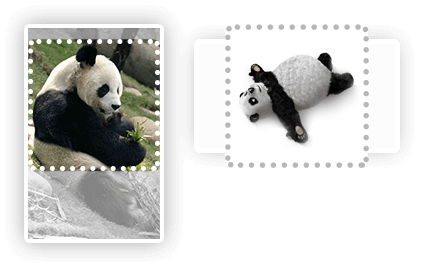
thumb
-
A more advanced implementation of cover that also detects cut out
images with plain backgrounds. The image is scaled down to the
width
andheight
you provide. If an image is detected with a free standing object it will add more background space where necessary or crop the unimportant parts. This feature is new and we’d love to hear your feedback!
If the target dimensions are larger than the original dimensions, the image will not be scaled up. Scaling up is prevented in order to protect the quality of your images.
Converting images
You can use the API to convert your images to your desired image type. Tinify currently supports converting between AVIF, WebP, JPEG, and PNG. When you provide more than one image type in your convert request, the smallest version will be returned to you.
Image converting will count as one additional compression.
Example convert request
POST /output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"convert": { "type": "image/webp" }
}
HTTP/1.1 200 OK
Compression-Count: 1
Content-Type: image/webp
Image-Width: 530
Image-Height: 300
Content-Length: 46480
[binary]
For example, to save a compressed PNG or JPEG image as a WebP file named panda-sticker.webp
:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--header "Content-Type: application/json" \
--data '{ "convert": { "type": "image/webp"} }' \
--dump-header /dev/stdout \
--output panda-sticker.webp
Example convert and transform request
If you wish to convert an image with a transparent background to one with a solid background,
specify a background
property in the transform
object. If this property is provided,
the background of a transparent image will be filled.
POST /output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"convert" : {
"type": "image/jpeg"
},
"transform": {
"background": "#000000"
}
}
HTTP/1.1 200 OK
Compression-Count: 1
Content-Type: image/jpeg
Image-Width: 530
Image-Height: 300
Content-Length: 46480
[binary]
For example, if you have an image with a transparent background and you want to save it as JPEG:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--header "Content-Type: application/json" \
--data '{ "convert" : {"type": "image/jpeg"}, "transform": {"background": "#000000"}}' \
--dump-header /dev/stdout \
--output panda-sticker.jpg
Request options
You can provide the following options to convert your image:
convert
-
Your desired image types. The following options are available as a
type
:-
One image type, specified as a string
"image/webp"
. -
Multiple image types, specified as an array
["image/webp","image/png"]
. The smallest of the provided image types will be returned. -
The wildcard
"*/*"
returns the smallest of Tinify's supported image types, currently AVIF, WebP, JPEG and PNG.
-
One image type, specified as a string
transform
-
The transform object specifies the stylistic transformations that will be applied to your image.
Include a
background
property to fill a transparent image's background. The following options are available to specify a background color:-
A hex value. Custom background color using the color's hex value:
"#000000"
. -
"white"
or"black"
. Only the colors white and black are supported as strings.
-
A hex value. Custom background color using the color's hex value:
Preserving metadata
You can request that specific metadata is copied from the uploaded image
to the compressed version. Preserving copyright
information, the GPS
location
and the creation
date are currently supported. Preserving
metadata adds to the compressed file size, so you should only preserve
metadata that is important to keep.
Preserving metadata will not count as an extra compression. However, in the background the image will be created again with the additional metadata.
Example upload request
You need to upload the original image first, similar to the previous examples.
POST /shrink HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
[binary]
HTTP/1.1 201 Created
Compression-Count: 1
Location: https://api.tinify.com/output/2xnsp7jn34e5
Content-Type: application/json
{
"output": {
"size": 45974,
"type": "image/jpeg"
}
}
For example, if you have a file named unoptimized.jpg
in the current folder:
curl https://api.tinify.com/shrink \
--user api:YOUR_API_KEY \
--data-binary @unoptimized.jpg \
--dump-header /dev/stdout
Example download request with metadata
You can now download the compressed version of the image with the
copyright information and creation date. Specify the URL that was
returned in the Location
header from the previous step and add the
JSON instructions to preserve
the copyright and creation date as
follows.
POST /output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"preserve": ["copyright", "creation"]
}
HTTP/1.1 200 OK
Compression-Count: 1
Content-Type: image/jpeg
Image-Width: 530
Image-Height: 300
Content-Length: 46480
[binary]
Notice that the Content-Length
will be slightly larger than the output
size
from the first step.
The following example shows how you can save the compressed image to a
file named optimized-copyright.jpg
where the copyright metadata has
been preserved.
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--header "Content-Type: application/json" \
--data '{ "preserve": ["copyright", "creation"] }' \
--dump-header /dev/stdout \
--output optimized-copyright.jpg
Request options
You can provide the following options to preserve specific metadata. No metadata will be added if the requested metadata is not present in the uploaded image.
copyright
- Preserves any copyright information. This includes the EXIF copyright tag (JPEG), the XMP rights tag (PNG) as well as a Photoshop copyright flag or URL. Uses up to 90 additional bytes, plus the length of the copyright data.
creation
- Preserves any creation date or time. This is the moment the image or photo was originally created. This includes the EXIF original date time tag (JPEG) or the XMP creation time (PNG). Uses around 70 additional bytes.
location
(JPEG only)- Preserves any GPS location data that describes where the image or photo was taken. This includes the EXIF GPS latitude and GPS longitude tags (JPEG). Uses around 130 additional bytes.
Saving to Amazon S3
You can ask the Tinify API to save compressed images straight to your Amazon S3. This feature is useful if you use S3 for images hosting, eliminating the need to download them to your server and manually upload them to S3. Each upload will be counted as an additional compression.
Example S3 request
You can store a compressed image to S3 by using the URL that was
returned in the Location
header. A JSON body with the bucket
information and a Content-Type: application/json
header have to be
included in the request that instructs the file to be stored on S3.
POST output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"store" : {
"service": "s3",
"aws_access_key_id": "AKIAIOSFODNN7EXAMPLE",
"aws_secret_access_key": "wJalrXUtnFEMI/K7MDENG/bPxRfiCYEXAMPLEKEY",
"region": "us-west-1",
"path": "example-bucket/my-images/optimized.jpg"
}
}
HTTP/1.1 200 OK
Compression-Count: 3
Image-Width: 530
Image-Height: 300
Location: https://s3-us-west-1.amazonaws.com/example-bucket/my-images/optimized.jpg
For example, to save the compressed image to a file named
my-images/optimized.jpg
in a bucked named example-bucket
:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--dump-header /dev/stdout --silent \
--header "Content-Type: application/json" \
--data '{
"store" : {
"service": "s3",
"aws_access_key_id": "AKIAIOSFODNN7EXAMPLE",
"aws_secret_access_key": "wJalrXUtnFEMI/K7MDENG/bPxRfiCYEXAMPLEKEY",
"region": "us-west-1",
"headers": {
"Cache-Control": "public, max-age=31536000"
},
"path": "example-bucket/my-images/optimized.jpg"
}
}'
Request options
You need to provide the following options in order to save an image on Amazon S3:
service
-
Specify
s3
to store to Amazon S3. aws_access_key_id
aws_secret_access_key
- Your AWS access key ID and secret access key. These are the credentials to an Amazon AWS user account. Find out how to obtain them in Amazon’s documentation. The user must have the correct permissions, see below for details.
region
- The AWS region in which your S3 bucket is located.
path
-
The path at which you want to store the image including the bucket
name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
.
The following settings are optional:
headers
(experimental)-
You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs.
acl
(optional)-
Set an optional Access Control List (ACL) value to manage object accessibility.
By default, objects are set to
public-read
. Useno-acl
to prevent sending an ACL. For more information about ACL options, refer to the AWS Canned ACL documentation.
service
-
Specify
gcs
to store to Google Cloud Storage. gcp_access_token
- The access token for authenticating to Google's Cloud Platform. Find out how to generate these tokens with the example above.
path
-
The path at which you want to store the image including the bucket
name. The path must be supplied in the following format:
<bucket>/<path>/<filename>
. headers
(experimental)-
You can add a
Cache-Control
header to control browser caching of the stored image, with for example:public, max-age=31536000
. The full list of directives can be found in the MDN web docs.
The user that corresponds to your AWS access key ID must have the
PutObject
and PutObjectAcl
permissions on the paths of the objects you
intend to create.
Example S3 access policy
If you want to create a user with limited access specifically for the Tinify API, you can use the following example policy as a starting point:
{
"Statement": {
"Effect": "Allow",
"Action": [
"s3:PutObject",
"s3:PutObjectAcl"
],
"Resource": [
"arn:aws:s3:::example-bucket/*"
]
}
}
Saving to Google Cloud Storage
You can tell the Tinify API to save compressed images directly to Google Cloud Storage. If you use GCS to host your images this saves you the hassle of downloading images to your server and uploading them to GCS yourself. Each upload will be counted as an additional compression.
Before you can store an image in GCS you will need to generate an access token with a service account.
Generating GCP access tokens
Please choose a language in the top of the page for the example code how to generate a new access token.
Example GCS request
You can store a compressed image to GCS by using the URL that was
returned in the Location
header. A JSON body with the bucket
information and a Content-Type: application/json
header have to be
included in the request that instructs the file to be stored on GCS.
POST output/2xnsp7jn34e5 HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
Content-Type: application/json
{
"store" : {
"service": "gcs",
"gcp_access_token": "EXAMPLE_TOKEN_Ag_0HvsglgriGh5_dqDqjaoGU_EXAMPLE_TOKEN",
"path": "example-bucket/my-images/optimized.jpg"
}
}
HTTP/1.1 200 OK
Compression-Count: 3
Image-Width: 530
Image-Height: 300
Location: https://storage.googleapis.com/example-bucket/my-images/optimized.jpg
For example, to save the compressed image to a file named
my-images/optimized.jpg
in a bucked named example-bucket
:
curl https://api.tinify.com/output/2xnsp7jn34e5 \
--user api:YOUR_API_KEY \
--dump-header /dev/stdout --silent \
--header "Content-Type: application/json" \
--data '{
"store" : {
"service": "gcs",
"gcp_access_token": "EXAMPLE_TOKEN_Ag_0HvsglgriGU_EXAMPLE_TOKEN",
"headers": {
"Cache-Control": "public, max-age=31536000"
},
"path": "example-bucket/my-images/optimized.jpg"
}
}'
Request options
You need to provide the following options in order to save an image on Google Cloud Storage:
The following settings are optional:
Saving to S3 compatible storage
Although the TinyPNG API does not offer an integrated solution for saving the images directly to S3 compatible storage providers other than Amazon, you can still save your images using the example for your chosen development language.
The S3 API is used by storage providers like Digital Ocean Spaces, Backblaze, G-Core Storage, Wasabi, Century Link Object Storage, Filebase and many others.
Please choose a language in the top of the page for the example code.
The detailed documentation on how to use the S3 API can be found here.
Saving to Microsoft Azure
Although the TinyPNG API does not offer an integrated solution for saving the images directly to Microsoft Azure storage, you can still save your images using the example for your chosen development language.
Please choose a language in the top of the page for the example code.
The detailed documentation on how to use the Azure storage API can be found here.
Error handling
The Tinify API uses HTTP status codes to indicate success or failure. Status codes in the 2xx range indicate success. Status codes in the 4xx range indicate there was a problem with your request. Status codes in the 5xx indicate a temporary problem with the Tinify API.
Example error response
In case an error occurs, the HTTP body will contain the error details
formatted as JSON. The message
will contain a short human readable
description.
In case of a rate limit exceeded error, please add some delay to consecutive requests. Tinify has a generous rate limit to ensure a high quality of service to all clients.
POST /shrink HTTP/1.1
Host: api.tinify.com
HTTP/1.1 401 Unauthorized
Content-Type: application/json
{
"error": "Unauthorized",
"message": "Credentials are invalid"
}
Compression count
The web service keeps track of the number of compressions you have made. Most HTTP responses include the number of compressions made this month.
Example response
In most responses the Compression-Count
header is present, which
contains the number of compressions made with this account during the
current calendar month.
POST /shrink HTTP/1.1
Host: api.tinify.com
Authorization: Basic YXBpOmFiY2RlZmdoaWprbG1ub3BxcnN0dXZ3eHl6MDEyMzQ1
[binary]
HTTP/1.1 201 Created
Compression-Count: 12
[...]
Need help? Got feedback?
We’re always here to help, so if you’re stuck just drop us a note on support@tinify.com. It’s also the perfect place to send us all your suggestions and feedback.